Programming languages follow different paradigms, shaping how developers write and structure code. These paradigms influence the overall approach to problem-solving, guiding how programs are designed and executed.
As a hands-on software architect, understanding these paradigms is essential when selecting the right programming language for a project. Each paradigm has its own strengths and weaknesses, and the choice can significantly impact scalability, maintainability, and performance. A solid grasp of these concepts enables architects and developers to make informed decisions that align with project goals and technical requirements.
In this article, we’ll take a brief look at the different programming language paradigms and their practical applications in software development and the IT industry.
Imperative Programming
Imperative programming is a paradigm that focuses on explicitly defining the sequence of commands a computer must execute to complete a task. In other words, it emphasizes how to achieve a task step by step. This approach relies on state changes and makes use of constructs like loops, conditionals, and assignments.
Imperative programming has two main subtypes:
- Procedural programming organizes code into reusable procedures or functions, promoting modularity and re-usability. Languages like C follow this approach.
- Structured programming enforces a clear flow of control using loops and conditionals instead of arbitrary jumps, such as
goto
. Languages like Python and Java exemplify this style.
Due to its straightforward and predictable execution model, imperative programming is widely used in system programming, scripting, and embedded systems.
Object-Oriented Programming
Object-oriented programming (OOP) is a coding approach that structures software around objects or instances of classes that bundle data and functionality together. It follows key principles like encapsulation, inheritance, and polymorphism, making code more modular. Because of its maintainability, OOP is widely used in application development, game design, and enterprise software. Popular languages like Java, C++, Python, and C# are built heavily around this concepts.
OOP enhances code organization by modeling real-world entities through classes and objects. Encapsulation helps protect data by controlling access to an object’s properties, while inheritance promotes code reuse by allowing new classes to build upon existing ones. Polymorphism enables flexible and dynamic execution, making OOP particularly effective for large-scale software projects.
Functional Programming
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions, avoiding changes to state and mutable data. It emphasizes pure functions, immutability, and minimizing side effects, making code more predictable, maintainable, and easier to reason about. Languages like Haskell, and Scala have long embraced functional programming, but its influence is now widespread, especially with the rise of frameworks like React in JavaScript.
One of functional programming’s biggest strengths is its natural ability to handle concurrency since immutable data structures eliminate race conditions. Features that can make it particularly well-suited for data transformation, distributed computing, and artificial intelligence are:
- Higher-Order Functions
- Lazy Evaluation
- Recursion
While functional programming was once considered niche, its principles have significantly shaped modern programming. Many mainstream languages, including JavaScript, Python, and Java, now incorporate functional features like map
reduce
, and lambda functions, making functional programming concepts more accessible than ever.
Declarative Programming
Declarative programming focuses on describing the desired outcome rather than explicitly defining how to achieve it. This approach allows developers to write concise and readable code without managing low-level control structures. It is commonly used in SQL, Haskell and Prolog.
Unlike imperative programming, where the focus is on execution steps, declarative programming shifts the responsibility to the underlying system. This paradigm is useful in following use-cases:
- Query Languages
- Configuration Management Like Terraform and Ansible
- UI Development like React
Since it abstracts away implementation details, declarative programming often leads to more maintainable and scalable code-bases.
Event-Driven Programming
Event-Driven Programming is a paradigm focused on responding to events such as user inputs, system triggers, or messages. It plays a key role in areas like graphical user interfaces , game development, and web applications. Languages such as JavaScript, C# with .NET event handlers, and Node.js are particularly well-suited for event-driven architectures.
At its core, an event-driven system continuously listens for events and executes the appropriate event handlers when triggered. This approach enables asynchronous execution, making applications more efficient and responsive. It is especially valuable in real-time applications, where prompt reactions to user interactions or external signals are essential.
Reactive Programming
Reactive Programming builds upon event-driven programming by emphasizing reactive data streams that automatically propagate changes throughout a system. This approach is particularly valuable for handling following cases:
- Asynchronous Data Processing
- Powering UI Frameworks
- Enabling Real-Time Applications.
Popular frameworks include RxJS (JavaScript), Reactor (Java), and Swift Combine.
Unlike traditional event-driven programming, which responds to individual events, reactive programming operates on continuous data streams that dynamically update over time. This makes it especially well-suited for highly responsive applications, such as financial systems, IoT solutions, and live data dashboards.
Concurrent & Parallel Programming
Concurrent Programming enables multiple tasks to make progress seemingly at the same time by interleaving their execution, while Parallel Programming executes tasks simultaneously across multiple CPU cores. Both paradigms are essential for optimizing performance in multithreading, distributed computing, and real-time systems.
Popular programming languages that support these approaches include Go, Java, and Python.
Concurrency enhances responsiveness by maximizing CPU utilization, whereas parallelism boosts computational speed by running independent tasks simultaneously. To manage the complexity of these approaches, developers rely on techniques such as thread pools, message passing, and task scheduling.
Wrapping Up
Each of the Programming Paradigms mentioned offers distinct approaches to problem-solving, making them suitable for different types of applications.
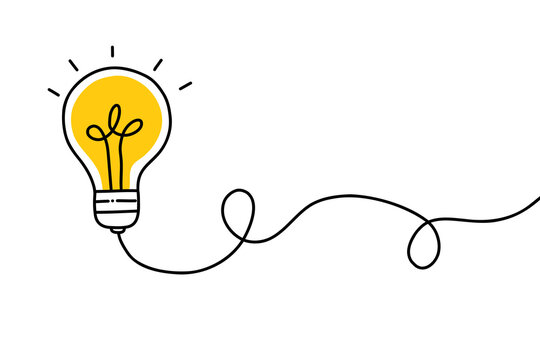
Imperative Programming provides a solid foundation with its step-by-step instructions, making it ideal for general-purpose and system-level tasks.
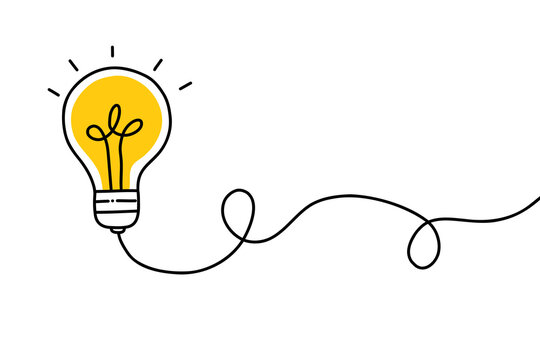
Object-Oriented Programming (OOP) introduces modularity and re-usability by organizing code around objects, which is particularly beneficial for large-scale software systems.
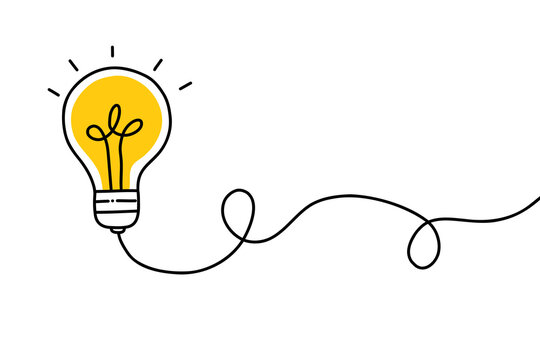
Functional Programming emphasizes immutability and pure functions, leading to more predictable and maintainable code, especially useful in data-intensive and concurrent systems.
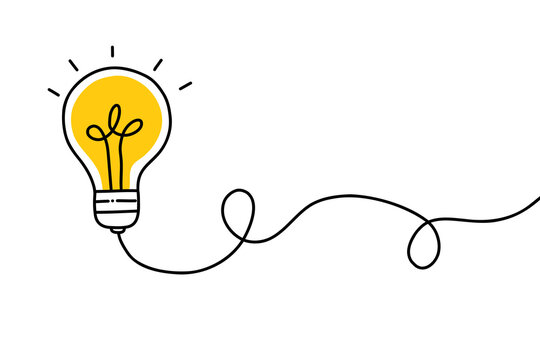
Declarative Programming simplifies tasks by focusing on what needs to be done rather than how it’s done.
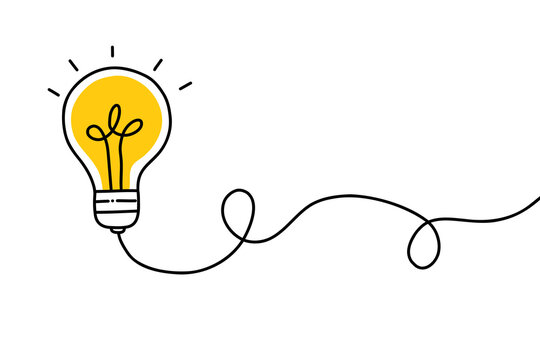
Event-Driven Programming thrives in dynamic, interactive applications like graphical user interfaces where it reacts to user inputs or system events.
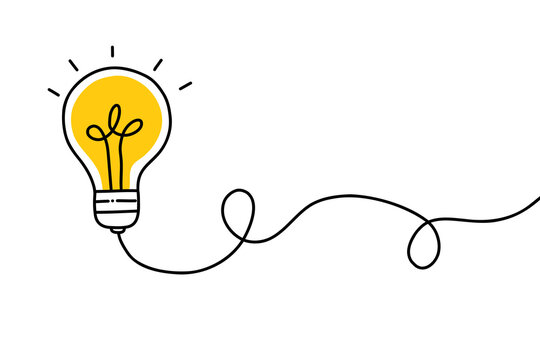
Reactive Programming builds on this by efficiently managing asynchronous data streams, making it an excellent choice for real-time applications.
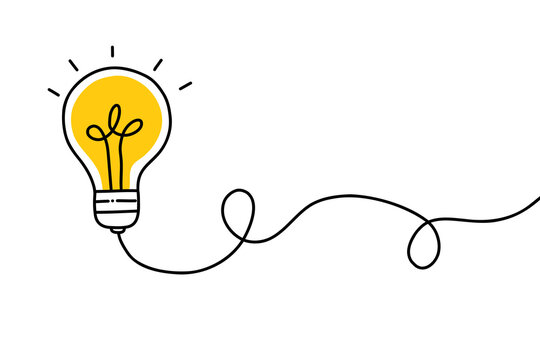
Concurrent & Parallel Programming optimize performance by running multiple tasks simultaneously, which is essential for applications requiring real-time processing.
Together, these paradigms provide developers with a versatile toolkit to tackle a wide variety of challenges in software design and performance optimization.
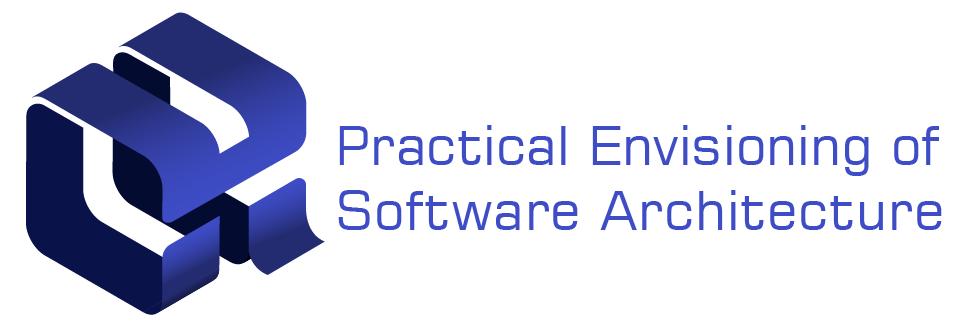