In modern software architecture, CQRS (Command Query Responsibility Segregation) and Event Sourcing are powerful patterns for building scalable and maintainable applications. These approaches fit well with Domain-Driven Design principles, making it easier to model complex business processes effectively.
CQRS and Event Sourcing help developers and architects design scalable, auditable systems that can easily adapt to future business changes. In this article, we’ll explore how these patterns work, their key benefits, and how to implement them effectively using a Domain-Driven Design approach.
Command Query Responsibility Segregation – CQRS
CQRS is an architectural pattern that separates read and write operations in a system, such as interactions with a database. Instead of using a single model to handle both queries (Reads) and commands (Writes), CQRS splits them into two distinct models, each optimized for its specific task as below:
- Command Model (Write Model)
- Query Model (Read Model)
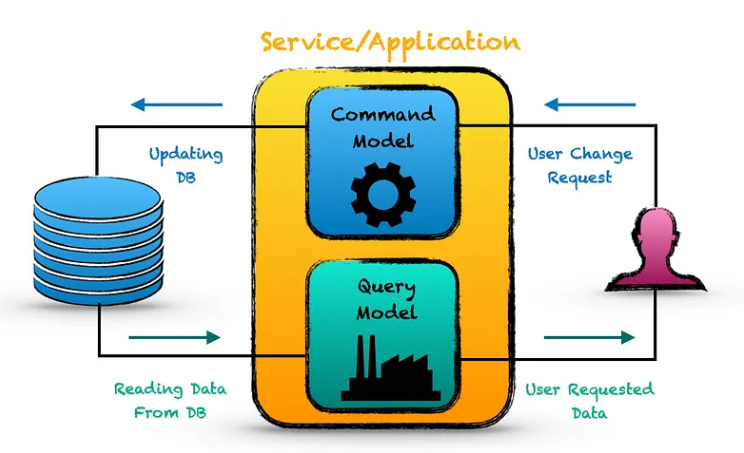
CQRS stands for Command Query Responsibility Segregation. It’s a pattern that I first heard described by Greg Young. At its heart is the notion that you can use a different model to update information than the model you use to read information.
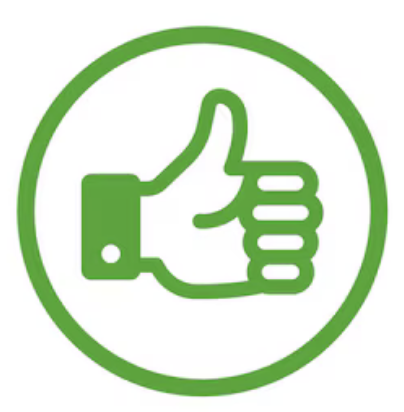
As an advantage, CQRS allows Independent Scaling of the command model and query model, optimizing performance for high-load applications. Furthermore, Performance Optimization is achievable by separately optimized queries with denormalized or cached views. By keeping the command and query sides separate, we can restrict who can modify data while allowing broader access for reading. This guarantees Security & Data Isolation.
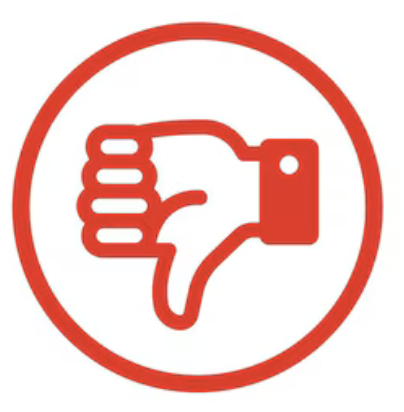
As disadvantages, maintaining two separate models in CQRS Increases Complexity which requires additional logic for synchronization. Furthermore, Since reads are updated asynchronously, there may be slight delays in data synchronization. This can result in temporarily outdated information, which is a common characteristic of Eventual Consistency.
Command Model
In CQRS, Command Model is responsible for handling operations that modify the application’s state, such as Create, Update and Delete operations in CRUD. This separation allows it to perform complex business logic without being tied to writing responsibilities. Additionally, it often interacts with a different persistence model than the query side.
Through the Command Model, operations such as rule validation, often governed by a policy engine, are executed to enforce domain-specific constraints and rules before any change is applied.
Query Model
This side of CQRS focuses on retrieving data and can be optimized independently from the write model. In fact, it often relies on denormalized data to speed up lookups.
As a complementary feature, the Read Model enhances scalability and performance by reducing the burden on the core domain model, allowing queries to be handled without disrupting ongoing transactions or command processes.
As summary, separating the command and query responsibilities into two distinct models in CQRS advocates for maintaining independent models for handling commands (which modify data) and queries (which retrieve data). Unlike the command model, which is the sole model responsible for making changes in the system, multiple read models can exist, each tailored to specific query needs.
Event Sourcing
Event Sourcing is an architectural pattern that records every change in an application as a sequence of immutable events, rather than simply updating a database.
Unlike traditional CRUD-based systems that only store the latest state, Event Sourcing keeps a history of all changes. This means the current state can always be reconstructed by replaying the recorded events in sequence.
Event Sourcing ensures that all changes to application state are stored as a sequence of events.
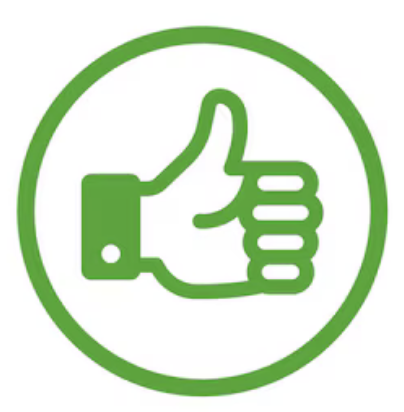
As advantages, event sourcing supports Auditability & Traceability with keeping a complete history of all changes in system. Furthermore, It guaranties Scalability by processing events asynchronously. It is also ideal for distributed systems. Last but not least, the system allows for Data Recovery & Debugging enabling you to restore it to any previous state whenever needed.
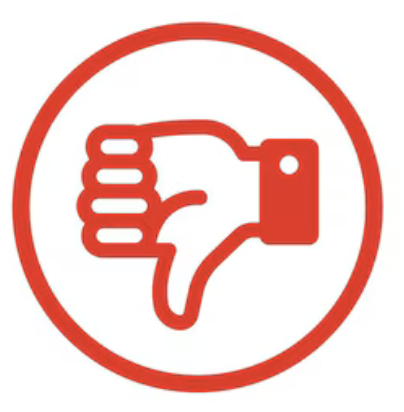
Complexity is one of the disadvantages of event sourcing architectural pattern. In fact, storing events, replaying them, and dealing with failures takes extra effort and careful management. High number of events can slow down the system over time. So, Handling Large Data Volumes could be tricky. Finally, If the Schema of Events changes, older events need to be managed properly to maintain consistency.
How Does It work?
Every Event Sourcing process can be summarized in three main steps:
- Every change in the system is recorded as a immutable event.
- Instead of modifying database records directly, events are stored in an “Event Store.”
- The system’s state is reconstructed by replaying these events.
Sample Code
To gain better understanding of CQRS and Event Sourcing combination in action, take a look at the provided simple example in this repository.
Wrapping Up
CQRS (Command Query Responsibility Segregation) and Event Sourcing are two architectural patterns that work well together, helping to build scalable, auditable, and domain-driven systems.
- CQRS separates the logic for writing (commands) and reading (queries) into distinct models. This separation makes it easier to optimize each operation independently and scale them as needed.
- Event Sourcing, on the other hand, records all changes in the system as a series of immutable events, preserving the complete history of state transitions. This makes it possible to reconstruct past states and gain a clear audit trail of what happened and when.
In the world of Domain-Driven Design, these two patterns naturally align with core principles. DDD encourages modeling business concepts as domain events, which fits perfectly with Event Sourcing. Meanwhile, CQRS enhances this approach by allowing separate models for handling commands and queries, reducing unnecessary complexity in a single data model.
Together, CQRS and Event Sourcing provide a solid foundation for building robust applications.
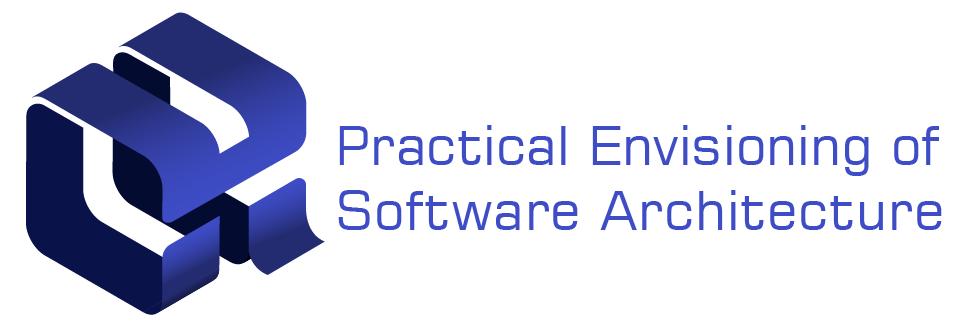