Modern software systems usually consist of various services, databases, and external integrations, which are often spread across different platforms and environments. APIs (Application Programming Interfaces) act as the connection that links all these parts, allowing them to work together and share data easily.
When designing APIs, several popular architectural styles are commonly considered as below:
- REST (REpresentational State Transfer)
- GraphQL
- gRPC (Google Remote Procedure Call)
Each has its own strengths and weaknesses, making it suitable for different use cases. Below, we compare them in terms of structure, performance, flexibility, and typical use cases. In addition to the various architectural styles, it’s important to consider key factors such as scalability, security, and integration ease when selecting the right API for your project. Let’s examine each of them briefly.
REST API
REST API (REpresentational State Transfer Application Programming Interface) is a way for applications to communicate with each other over the web using a set of standardized rules. It follows the REST architectural style, which was introduced by Roy Fielding in his 2000 dissertation. REST APIs are designed to be stateless, scalable, and flexible, allowing seamless data exchange between systems. However, they typically operate in a synchronous manner.
REST APIs play n important role in modern software development, powering web and mobile applications, enabling microservices to communicate, supporting IoT devices, and facilitating data sharing and third-party integrations.
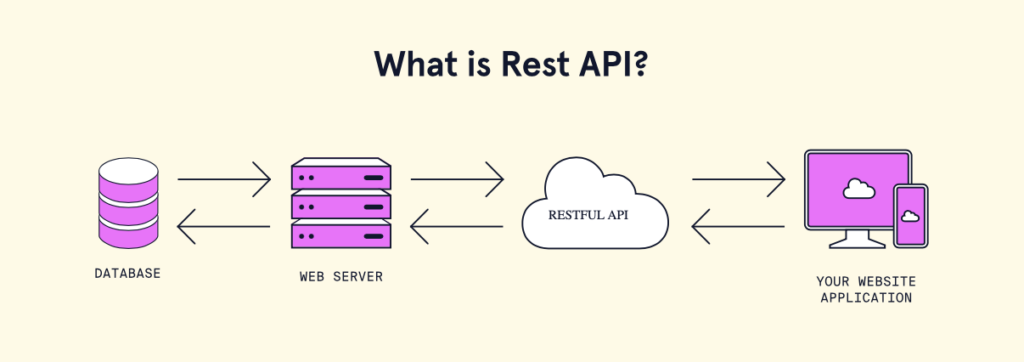
How REST API Works?
When a client application, like a web browser and mobile app, connects with a REST API, here’s what happens:
- The client sends an HTTP request to a specific API endpoint.
- The server processes the request, often retrieving data from a database or sometimes running some business logic.
- The server responds with the requested data or a status message, letting the client know what happened.
Features
In the table below, you’ll find the key characteristics of REST APIs.
Client-Server Architecture | In this architectural style, clients (consumer) request and receive service from a server (producer). |
Statelessness | The server doesn’t retain memory or store any client-specific session data between requests. That’s why every API request must include all the necessary information for the server to process it. |
Cache-ability | Responses from a REST API can be cached to enhance performance and reduce server load, often using techniques like Cache-Control headers. |
Uniform Interface (HTTP Based) | REST APIs has a consistent and standardized way of communication based on HTTP protocol. GET – Retrieve data, POST – Create new data, PUT – Update existing data and DELETE – Remove data |
Layered Design | Client only interacts with the designated endpoints. Furthermore, REST APIs have multiple layers, such as security, load balancing, caching and so on, which allows flexibility in deployment. |
GraphQL
GraphQL is a powerful query language for APIs and a runtime for running those queries. Originally developed by Facebook in 2012 and open-sourced in 2015, it offers a more flexible and efficient alternative to REST API. With GraphQL, clients can request precisely the data they need, nothing more, nothing less, helping to eliminate issues like over-fetching and under-fetching of data.
How GraphQL Works?
When a client app communicates with a GraphQL API, here’s what happens:
- The app sends a request with a GraphQL query to the API endpoint (usually
/graphql
). - The GraphQL server reads the query and checks the schema to figure out what data to retrieve.
- The server responds with exactly the requested data, typically in JSON format.
Here you can see a simple example of query/response pair.
query {
user(id: a490) {
name
email
orders {
id
status
}
}
}
{
"data": {
"user": {
"name": "John Doe",
"email": "john.doe@example.com",
"orders": [
{
"id": "A1B2C3",
"status": "Shipped"
}
]
}
}
}
Features
In the table below, you’ll find the key characteristics of GraphQL.
Declarative Query Language | Clients can define the precise shape and structure of the data they need in a single query. This not only ensures they get exactly what they’re looking for but also minimizes unnecessary data transfer. |
Single Endpoint | GraphQL APIs provide a single endpoint (e.g., /graphql ), where the client specifies exactly what data it needs in the query. This is different from REST, which relies on multiple endpoints for different types of data. |
Flexible Data Fetching | It lets clients customize their response structure, ensuring they get exactly the data they need, neither over-fetching, nor under-fetching. |
Hierarchical Data Retrieval | GraphQL queries can fetch related data in a single request. |
Real-time Data with Subscriptions | It supports real-time updates through subscriptions, making it great for live chat apps, notifications, and streaming data. |
gRPC
gRPC (Remote Procedure Call) is an open-source framework from Google that helps services communicate quickly and efficiently. Instead of using JSON or XML, it relies on Protocol Buffers (Protobuf) for communication and data exchange, which makes it faster and more lightweight.
Protocol Buffers are language-neutral, platform-neutral extensible mechanisms for serializing structured data.1
Built for speed and scalability, gRPC is widely used in cloud-native applications, real-time systems, and distributed architectures where efficient communication between microservices is essential.
Like many RPC systems, gRPC is based around the idea of defining a service, specifying the methods that can be called remotely with their parameters and return types.2
How gRPC Works?
To use the gRPC protocol when a client application (like a microservice) communicates with a server, here’s how it works:
- Define the API with Protocol Buffers: A
.proto
file defines the service, methods, request, and response types. - Compile the
.proto
File to Generate Code: This step generates client and server code in multiple languages. - Implement and Deploy gRPC server Logic: It implements the service methods defined in the
.proto
file and handles tasks such as business logic, connects to a database, and returns responses. - Implement and Deploy Clients: Clients call remote functions directly using the generated code.
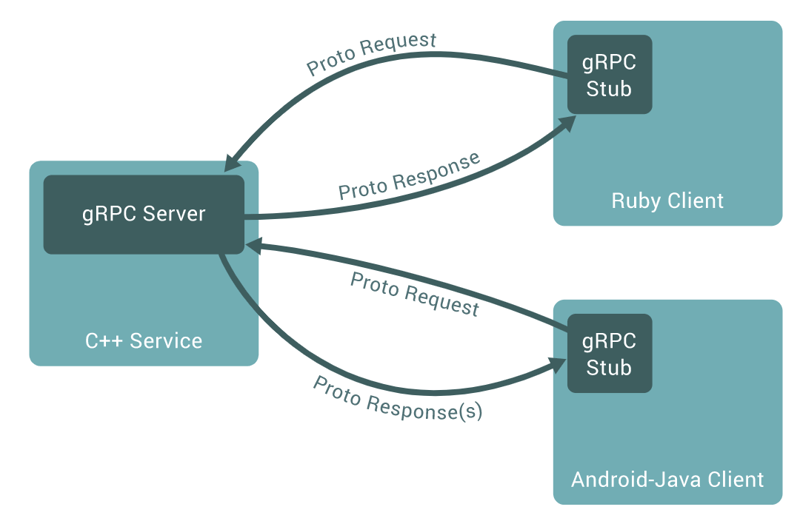
Features
In the table below, you’ll find the key characteristics of gRPC.
Uses Remote Procedure Calls (RPC) | Unlike REST, where clients send HTTP requests to specific endpoints, gRPC allows clients to call functions on remote servers just like they would call local functions. |
Built-in Authentication & Security | gRPC offers strong security features, including TLS encryption, authentication methods like OAuth and JWT, and mTLS for added protection. |
Serialization via Protobuf | gRPC uses Protocol Buffers (Protobuf), a lightweight and efficient binary format. |
Multiple Communication Types Support | It supporst Unary RPC (like REST), Server Streaming, Client Streaming and Bidirectional Streaming (Both client and server send multiple messages) |
High Performance & Low Latency | gRPC is optimized for high-performance communication, making it faster than other APIs. Furthermore, lower network overhead due to binary serialization with Protobuf. |
REST API vs. GraphQL vs. gRPC
In this section, we compare these API solutions from various perspectives, including data format, schema, security, and other relevant factors.
Protocol
- REST API: A simple protocol based on HTTP that lets clients and servers communicate using basic methods like
GET
,POST
,PUT
, andDELETE
. - GraphQL: A flexible way to query APIs, allowing clients to ask for exactly the data they need in one go, instead of multiple requests.
- gRPC: A fast and efficient communication protocol based on HTTP/2, using Protocol Buffers to quickly send data between services.
Data Format & Schema
- REST API: Usually uses JSON or XML to send and receive data between the client and server with flexible schema.
- GraphQL: Uses JSON for both sending requests and getting responses, with the ability to fetch only the data you need and strongly types schema.
- gRPC: Uses Protocol Buffers (Protobuf), a fast and compact binary format, to communicate between systems which is the strict schema.
Security
- REST API: Uses standard security methods like SSL/TLS, OAuth, and API keys to protect communication.
- GraphQL: Secures communication through HTTP and can also use extra protections like query whitelisting, OAuth, and role-based access control for endpoint security.
- gRPC: Protects communication with SSL/TLS encryption and supports token-based authentication and client-side certificate validation for added security and mTLS.
Use cases
Here’s a more straightforward, humanized version:
- REST API: Great for simple tasks like CRUD (creating, reading, updating, or deleting) data, especially when dealing with public APIs or web services that don’t require keeping track of state.
- GraphQL: Perfect for apps that need flexible, efficient data fetching, especially when dealing with complex relationships or lots of nested data (like modern websites or mobile apps).
- gRPC: Ideal for fast, real-time communication between services, especially when handling large amounts of data or low-latency needs.
Wrapping Up
API architecture is essential in today’s software world because it gives a clear and organized way for different software components to communicate. As technologies like microservices, cloud computing, and distributed systems become more common, APIs help use of solutions like Domain-Driven Design for software eco-systems.
REST API (Representational State Transfer) is the most widely used in software architecture. It uses HTTP protocols to handle requests and responses without keeping any memory of previous interactions.
GraphQL, created by Facebook, improves on REST by letting clients request exactly the data they need, instead of getting a fixed set of information.
On the other hand, gRPC is built for fast, low-latency communication, making it ideal for microservices where performance is crucial. Unlike REST API and GraphQL, which use text-based formats, gRPC uses protobufs, a more efficient binary format.
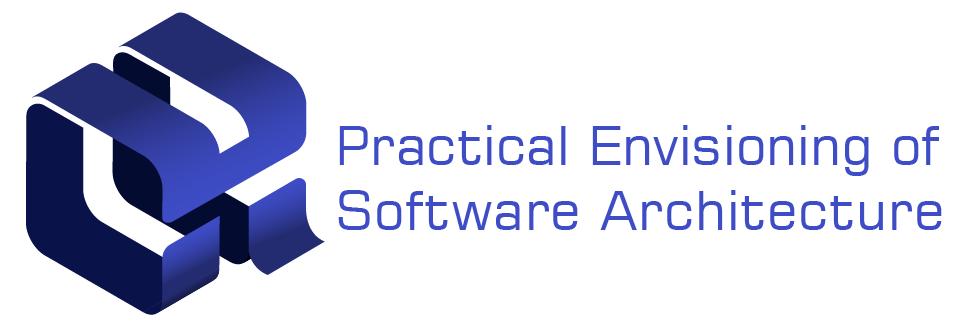